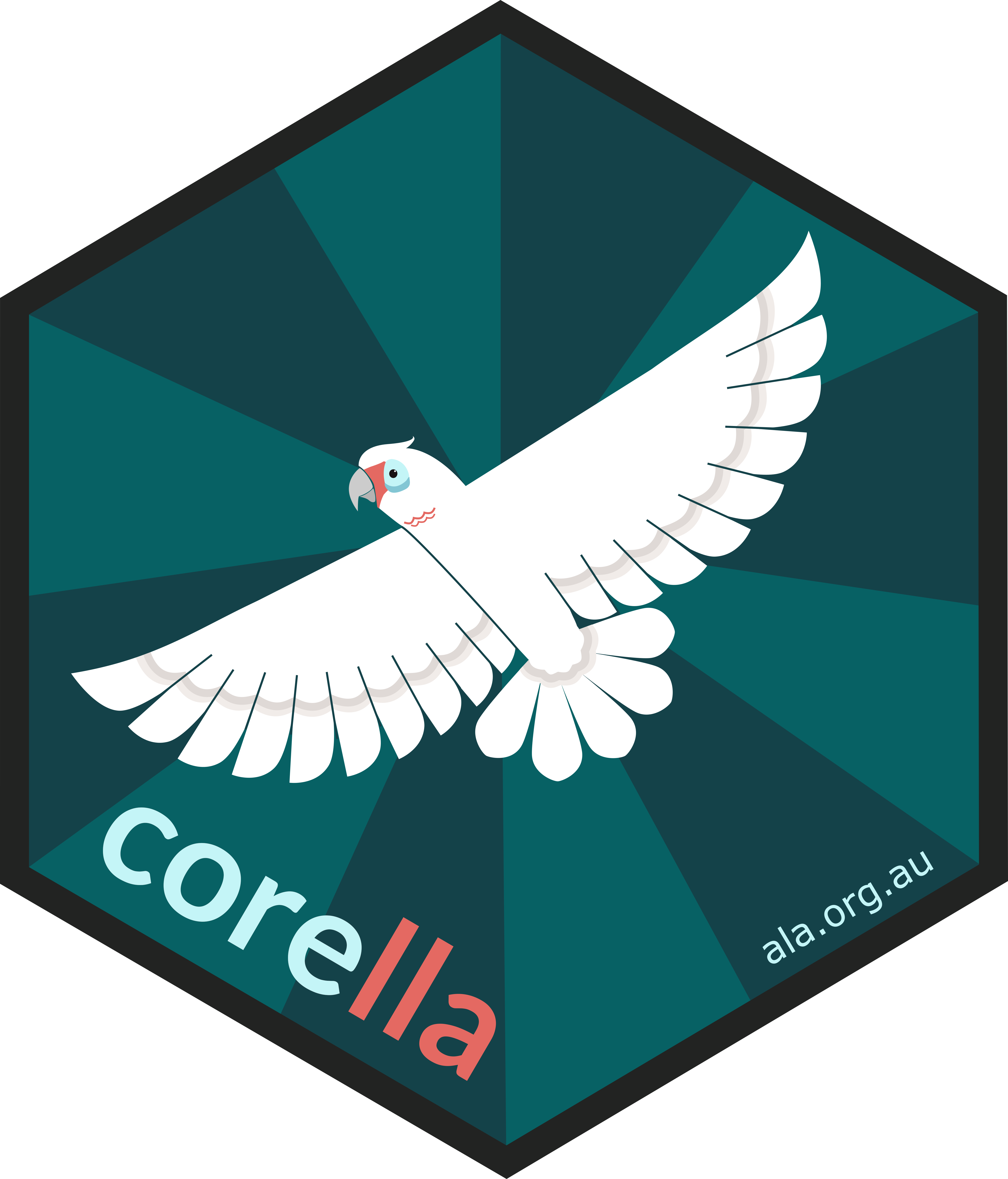
Set, create or modify columns with date and time information
Source:R/set_datetime.R
set_datetime.Rd
This function helps format standard date/time columns in a tibble
using
Darwin Core Standard. Users should make use of the
lubridate package to
format their dates so corella can read them correctly.
In practice this is no different from using mutate()
, but gives some
informative errors, and serves as a useful lookup for how spatial fields are
represented in the Darwin Core Standard.
Usage
set_datetime(
.df,
eventDate = NULL,
year = NULL,
month = NULL,
day = NULL,
eventTime = NULL,
.keep = "unused",
.messages = TRUE
)
Arguments
- .df
A
data.frame
ortibble
that the column should be appended to.- eventDate
The date or date + time that the observation/event occurred.
- year
The year of the observation/event.
- month
The month of the observation/event.
- day
The day of the observation/event.
- eventTime
The time of the event. Use this term for Event data. Date + time information for observations is accepted in
eventDate
.- .keep
Control which columns from .data are retained in the output. Note that unlike
dplyr::mutate()
, which defaults to"all"
this defaults to"unused"
; i.e. only keeps Darwin Core fields, and not those fields used to generate them.- .messages
(logical) Should informative messages be shown? Defaults to
TRUE
.
Details
Example values are:
eventDate
should be classDate
orPOSITct
. We suggest using the lubridate package to define define your date format using functions likeymd()
,mdy
,dmy()
, or if including date + time,ymd_hms()
,ymd_hm()
, orymd_h()
.
Examples
df <- tibble::tibble(
name = c("Crinia Signifera", "Crinia Signifera", "Litoria peronii"),
latitude = c(-35.27, -35.24, -35.83),
longitude = c(149.33, 149.34, 149.34),
date = c("2010-10-14", "2010-10-14", "2010-10-14"),
time = c("10:08:12", "13:01:45", "14:02:33")
)
# Use the lubridate package to format date + time information
# eventDate accepts date + time
df |>
set_datetime(
eventDate = lubridate::ymd_hms(paste(date, time))
)
#> ⠙ Checking 1 column: eventDate
#> ✔ Checking 1 column: eventDate [310ms]
#>
#> # A tibble: 3 × 4
#> name latitude longitude eventDate
#> <chr> <dbl> <dbl> <dttm>
#> 1 Crinia Signifera -35.3 149. 2010-10-14 10:08:12
#> 2 Crinia Signifera -35.2 149. 2010-10-14 13:01:45
#> 3 Litoria peronii -35.8 149. 2010-10-14 14:02:33