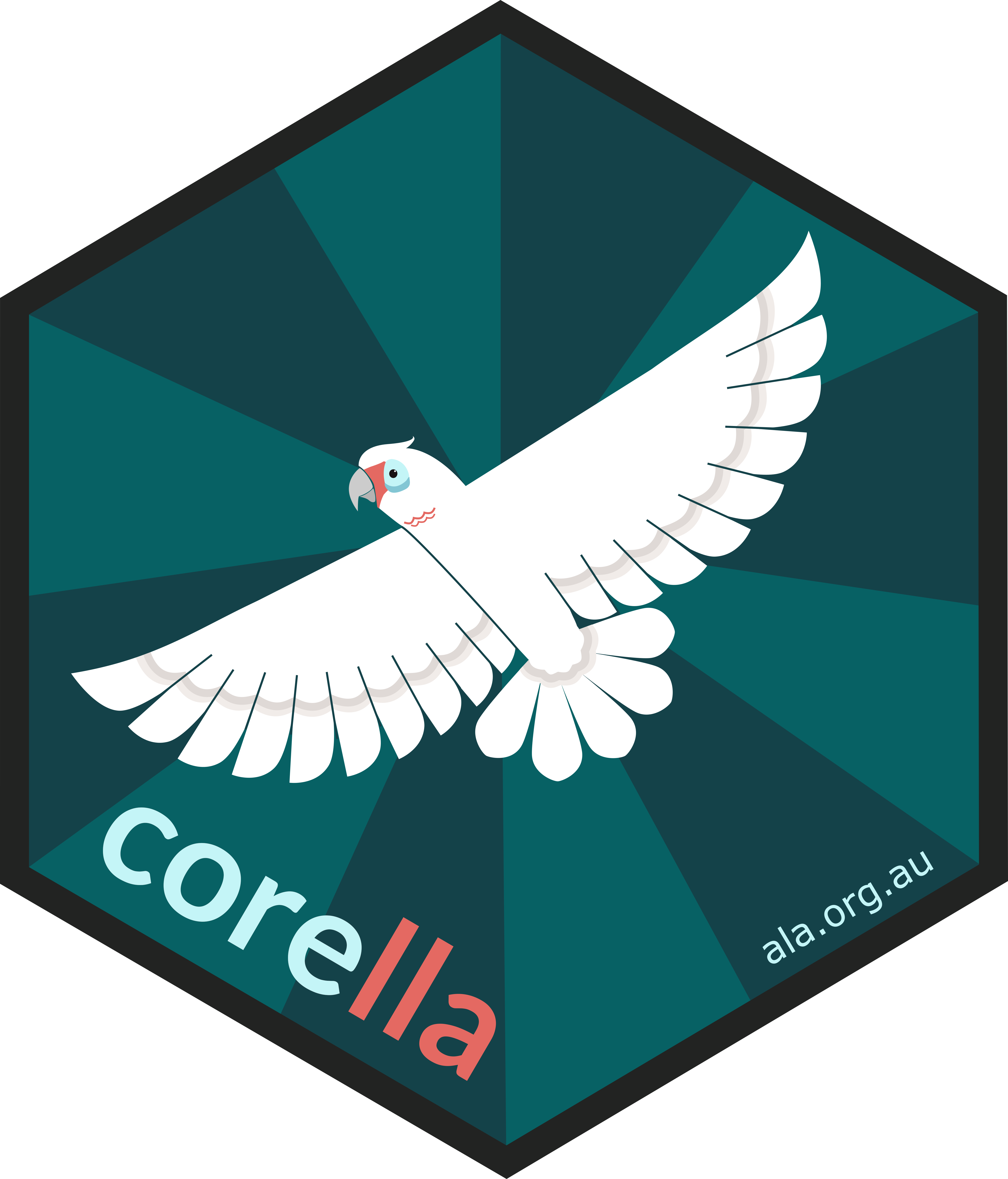
Convert columns with measurement data for an individual or event to Darwin Core standard
Source:R/set_measurements.R
set_measurements.Rd
This function is a work in progress, and should be used with caution.
In raw collected data, many types of information can be captured in one
column. For example, the column name LMA_g.m2
contains the measured trait
(Leaf Mass per Area, LMA) and the unit of measurement (grams per meter
squared, g/m2), and recorded in that column are the values themselves. In
Darwin Core, these different types of information must be separated into
multiple columns so that they can be ingested correctly and aggregated with
sources of data accurately.
This function converts information preserved in a single measurement column
into multiple columns (measurementID
, measurementUnit
, and
measurementType
) as per Darwin Core standard.
Arguments
- .df
a
data.frame
ortibble
that the column should be appended to.- cols
vector of column names to be included as 'measurements'. Unquoted.
- unit
vector of strings giving units for each variable
- type
vector of strings giving a description for each variable
- .keep
Control which columns from .data are retained in the output. Note that unlike
dplyr::mutate()
, which defaults to"all"
this defaults to"unused"
; i.e. only keeps Darwin Core fields, and not those fields used to generate them.
Details
Columns are nested in a
single column measurementOrFact
that contains Darwin Core Standard
measurement fields. By nesting three measurement columns within the
measurementOrFact
column, nested measurement columns can be converted to
long format (one row per measurement, per occurrence) while the original
data frame remains organised by one row per occurrence. Data
can be unnested into long format using tidyr::unnest()
.
Examples
if (FALSE) { # \dontrun{
library(tidyr)
# Example data of plant species observations and measurements
df <- tibble::tibble(
Site = c("Adelaide River", "Adelaide River", "AgnesBanks"),
Species = c("Corymbia latifolia", "Banksia aemula", "Acacia aneura"),
Latitude = c(-13.04, -13.04, -33.60),
Longitude = c(131.07, 131.07, 150.72),
LMA_g.m2 = c(NA, 180.07, 159.01),
LeafN_area_g.m2 = c(1.100, 0.913, 2.960)
)
# Reformat columns to Darwin Core Standard
# Measurement columns are reformatted and nested in column `measurementOrFact`
df_dwc <- df |>
set_measurements(
cols = c(LMA_g.m2,
LeafN_area_g.m2),
unit = c("g/m2",
"g/m2"),
type = c("leaf mass per area",
"leaf nitrogen per area")
)
df_dwc
# Unnest to view full long format data frame
df_dwc |>
tidyr::unnest(measurementOrFact)
} # }